BottomNavigationBar の高さを調整する方法をまとめていきます。
SizedBox で高さを指定する
@override
Widget build(BuildContext context) {
return Scaffold(
body: const Center(
child: Text('BottomNavigationBar'),
),
bottomNavigationBar: SizedBox(
height: 120,
child: BottomNavigationBar(
items: const [
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.search),
label: 'Search',
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
label: 'Settings',
),
],
backgroundColor: Colors.cyan,
)),
);
}
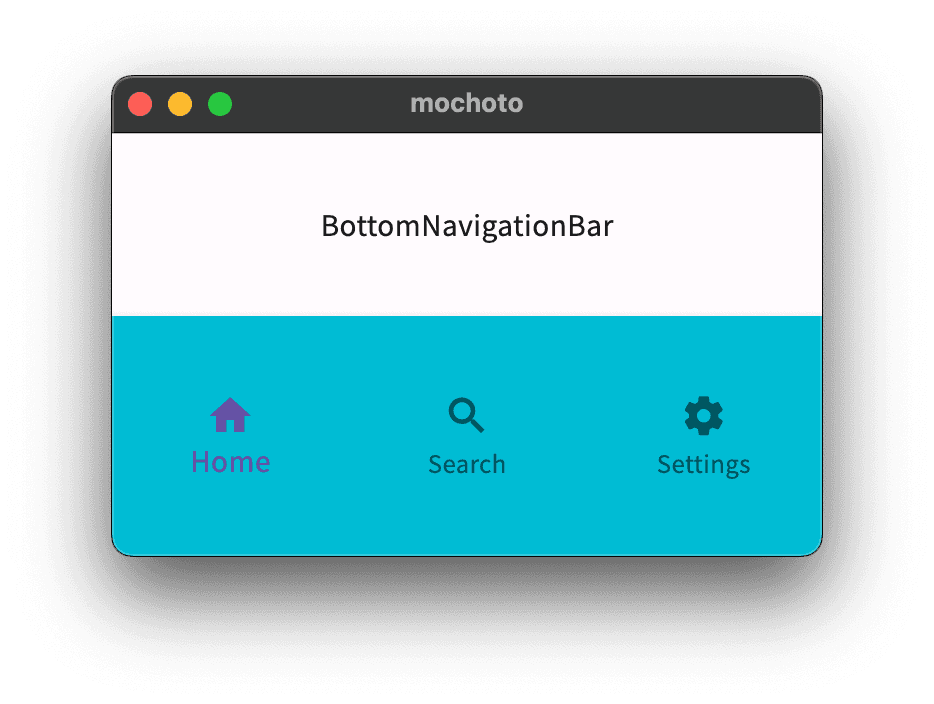
Container で高さを調整する
Container を利用し、height と padding で高さを調整しています。
@override
Widget build(BuildContext context) {
return Scaffold(
body: const Center(
child: Text('BottomNavigationBar'),
),
bottomNavigationBar: Container(
height: 100,
padding: const EdgeInsets.symmetric(vertical: 10),
decoration: const BoxDecoration(
color: Colors.cyanAccent,
),
child: BottomNavigationBar(
items: const [
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.search),
label: 'Search',
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
label: 'Settings',
),
],
elevation: 0,
backgroundColor: Colors.cyan,
)),
);
}
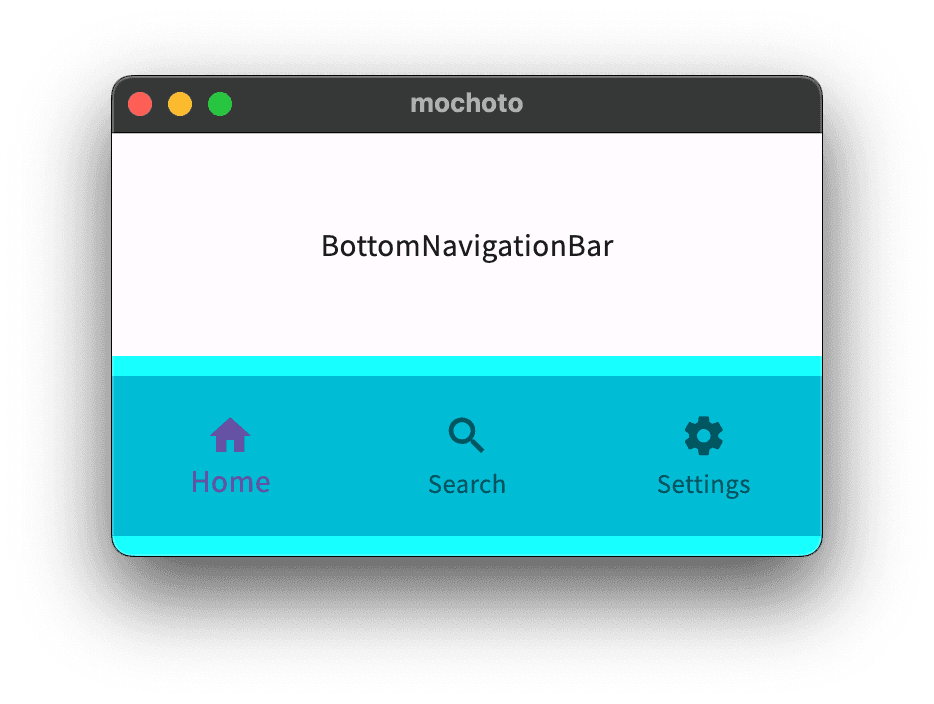
label を非表示にした場合の余白を調整する
Label を非表示にした場合に余分な余白ができてしまう場合は、フォントサイズを調整します。
selectedFontSize
と unselectedFontSize
を 0 に設定します。
@override
Widget build(BuildContext context) {
return Scaffold(
body: const Center(
child: Text('BottomNavigationBar'),
),
bottomNavigationBar: BottomNavigationBar(
showSelectedLabels: false,
showUnselectedLabels: false,
selectedFontSize: 0,
unselectedFontSize: 0,
items: const [
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.search),
label: 'Search',
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
label: 'Settings',
),
],
elevation: 0,
backgroundColor: Colors.cyan,
),
);
}
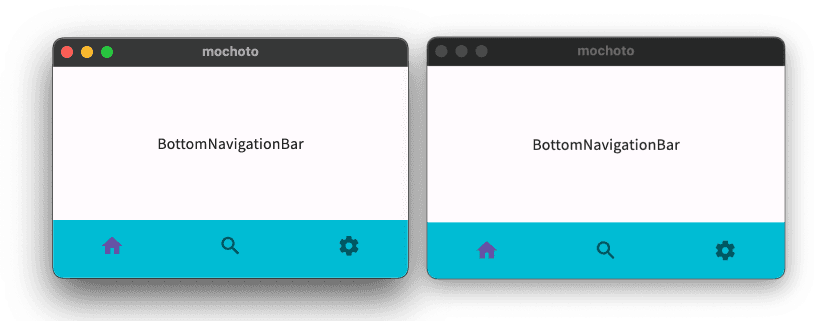