Flutter で WebView 表示をflutter_inappwebview パッケージで実装する機会がありましたので使い方をまとめていきます。
flutter_inappwebview は多機能で色々できますが、今回は指定した URL の Web ページを表示するInAppWebView
について書きます。
パッケージをインストール
flutter pub add flutter_inappwebview
dependencies:
flutter_inappwebview: ^6.0.0
WebView 内に Web サイトを表示
import 'package:flutter_inappwebview/flutter_inappwebview.dart';
でパッケージを import します。- main() で
WidgetsFlutterBinding.ensureInitialized();
を追加します。 - widget で
InAppWebView
を追加し,initialUrlRequest
に表示する URL を指定します。
lib/main.dart
import 'package:flutter/material.dart';
import 'package:flutter_inappwebview/flutter_inappwebview.dart'; // <-- 1
void main() {
WidgetsFlutterBinding.ensureInitialized(); // <-- 2
runApp(const MaterialApp(home: MyApp()));
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
final GlobalKey webViewKey = GlobalKey();
InAppWebViewController? webViewController;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text("Mochoto InAppWebView")),
body: SafeArea(
child: InAppWebView( // <-- 3
key: webViewKey,
initialUrlRequest: URLRequest(url: WebUri("https://inappwebview.dev/")),
onWebViewCreated: (controller) {
webViewController = controller;
},
)),
);
}
}
起動すると指定した URL の Web ページが表示されます。
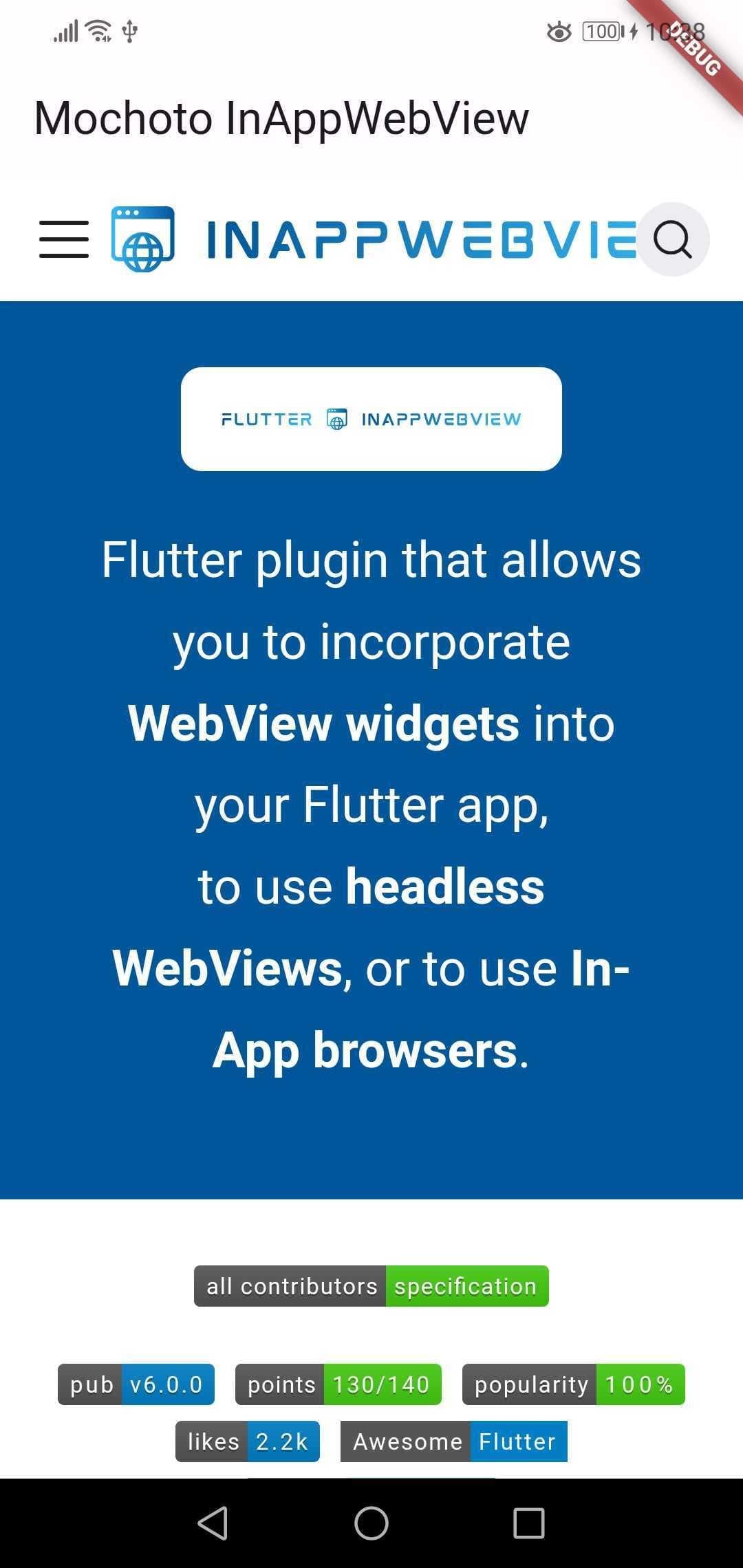
ビルドでExecution failed for task ':app:checkDebugAarMetadata'.
が出る場合
Requirements に合わせてandroid/app/build.gradle
のバージョンを変更します。
Getting Started | InAppWebView
InstallationcompileSdkVersion と targetSdkVersion を 34, minSdkVersionw を 19 に 変更しました。
android/app/build.gradle
android {
compileSdkVersion 34
・・・
defaultConfig {
・・・
minSdkVersion 19
targetSdkVersion 34
Web ページ の console ログを取得
Web ページ側で出力した console ログを取得する方法です。 InAppWebView のプロパティに onConsoleMessage 追加を追加し、consoleMessage で ログ内容を取得します。
lib/main.dart
InAppWebView(
key: webViewKey,
onConsoleMessage: (controller, consoleMessage) {
if (kDebugMode) {
print(consoleMessage);
}
},
web ページ側でconsole.log("mochoto web home");
を実行すると、以下のようにログが出力されます。
I/flutter ( 4210): ConsoleMessage{message: mochoto web home, messageLevel: LOG}
InAppWebView: The Real Power of WebViews in Flutter | InAppWebView
InAppWebView article logo
関連記事
[Flutter]WebviewでローカルホストとHTTP接続する
FlutterのWebview実装時にローカルホストのWebアプリと通信する方法です。![[Flutter]WebviewでローカルホストとHTTP接続する](https://www.mochot.com/opengraph-image.png)